How to Get the Current Directory in Python
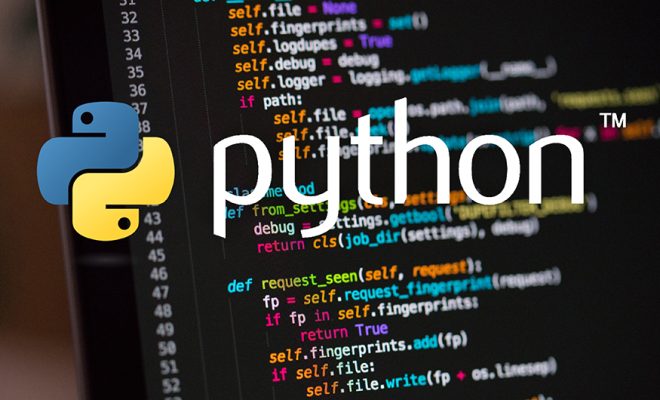
As a novice Python user, it is essential to know how to obtain the current working directory in Python. Understanding what a current directory is and how to get it helps programmers to locate and access file paths, use system resources effectively, and design clean and efficient code.
In Python, a current working directory (CWD) is a folder path where the Python script runs from. It is the directory where all the file operations like reading or writing files take place. When a Python script runs on a computer, it starts in the directory defined in the command prompt or terminal. Therefore, to find the CWD, we need to write Python code that fetches it.
Let us walk through three different ways of getting the CWD:
Method 1: Using the os Library
The first method of getting the CWD in Python is by using the os library. The os library in Python provides a cross-platform interface to execute system calls. It offers methods to retrieve information about the file system and the current working directory.
Here’s how to use this method:
– Import the os library: Write the following code at the beginning of your program
“`
import os
“`
– Get the current working directory: Use the os.getcwd() method to obtain the current directory.
“`
cwd = os.getcwd()
print(cwd)
“`
The above code returns a string that represents the current working directory of your Python program.
Method 2: Using the Pathlib Library
The second method of getting the CWD in Python is by using the pathlib library. It is a robust library that provides classes to handle file paths and directories. It is available in Python 3.4 and higher versions.
Here’s how to use this method:
– Import the pathlib library: Write the following code at the beginning of your program
“`
from pathlib import Path
“`
– Get the current working directory: Use the Path.cwd() method to obtain the current directory.
“`
cwd = Path.cwd()
print(cwd)
“`
The above code returns a Path object that represents the current working directory of your Python program.
Method 3: Using the os Path module
The third method of getting the CWD in Python is by using the os.path module. It is a sub-module of the os library with access to common path functionalities.
Here’s how to use this method:
– Import the os library: Write the following code at the beginning of your program
“`
import os
“`
– Get the current working directory: Use the os.path.abspath(os.getcwd()) to obtain the current directory.
“`
cwd = os.path.abspath(os.getcwd())
print(cwd)
“`
The above code returns a string that represents the current working directory of your Python program.