What Is the Factory Design Pattern?
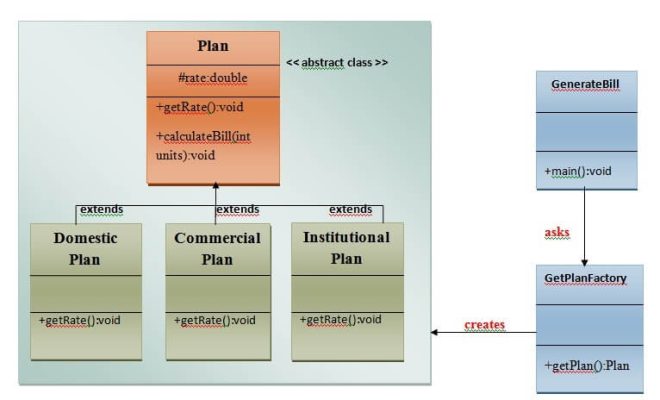
The Factory Design Pattern is a creational pattern that allows creating objects without the need to specify the exact class of object that will be created. In other words, a Factory Design Pattern hides the creation logic of an object and delegates the responsibility of creating objects to a factory.
This pattern is widely used in software engineering to create objects based on a set of parameters or conditions. It provides a common interface for creating different types of objects, which ensures that the client code remains unaltered even when new objects are added.
The Factory Design Pattern consists of the following key elements:
1. Factory: The factory is responsible for creating objects and returns the created object based on a set of conditions or parameters.
2. Product: The product is the object that the factory creates. It can be a simple, complex or abstract object.
3. Creator: The creator is an abstraction that defines the interface for creating objects. It can be an interface or an abstract class.
How does the Factory Design Pattern work?
The Factory Design Pattern works by creating a dedicated factory class that creates objects based on certain conditions or parameters. The factory class usually has a single method that is responsible for creating objects. This method accepts parameters that are used to determine the type of object to be created.
The client code accesses the factory method, which returns the created object without the need to know the implementation details of the creation process. This ensures that the client code remains loosely coupled with the object creation process and can be easily modified without affecting the rest of the system.
Benefits of using the Factory Design Pattern
The Factory Design Pattern has numerous benefits, including:
1. Code reusability: Since the Factory Design Pattern provides a common interface for creating different types of objects, it makes it easy to create new objects without having to rewrite code.
2. Simplified code: By abstracting the object creation process, the Factory Design Pattern makes the code simple and easy to read, understand and maintain.
3. Flexibility: The Factory Design Pattern allows for easy customization of the object creation process. By changing the factory class, it is possible to create different types of objects, and this makes the system flexible and adaptable to changing requirements.
Conclusion
The Factory Design Pattern is an essential element for software development. It ensures the separation of concerns between the object creation process and client code. With this pattern, it is easy to create new objects, customize the object creation process, and maintain the code quality. If you’re looking for a way to improve your software architecture, consider using the Factory Design Pattern.