What Does the Python enumerate() Function Do, and How Do You Use It?
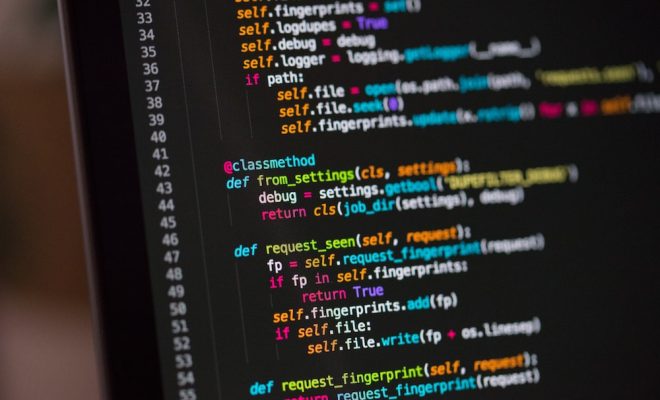
The Python programming language offers a wide range of built-in functions that simplify code and make it easier to manipulate data. One such function is the enumerate() function, which allows you to iterate over a sequence and simultaneously keep track of the current element index.
In this article, we will take an in-depth look at the Python enumerate() function, how it works, and how you can use it in your Python projects.
What is the Python enumerate() function?
The enumerate() function in Python is a built-in function that takes an iterable object, such as a list, tuple, or string, and returns an iterator object that generates tuples. These tuples contain the index and the corresponding item of the sequence it is iterating over.
The syntax of the Python enumerate() function is as follows:
“`
enumerate(sequence, start=0)
“`
Here, “sequence” refers to the iterable object you want to iterate over, while “start” is an optional argument that defines the starting value of the index variable. By default, the starting value of “start” is set to 0.
How to use the Python enumerate() function?
Now, let’s take a look at some examples of how to use the Python enumerate() function in your code.
Example 1: Using the enumerate() function to loop over a list
“`
fruits = [“apple”, “banana”, “cherry”, “date”]
for i, fruit in enumerate(fruits):
print(i, fruit)
“`
Output:
“`
0 apple
1 banana
2 cherry
3 date
“`
Here, the enumerate() function loops over the “fruits” list and generates tuples that contain an index and a value. The “i” variable holds the index value, while the “fruit” variable holds the corresponding value from the list.
Example 2: Using the enumerate() function to start counting from a specific number
“`
fruits = [“apple”, “banana”, “cherry”, “date”]
for i, fruit in enumerate(fruits, 1):
print(i, fruit)
“`
Output:
“`
1 apple
2 banana
3 cherry
4 date
“`
In this example, we are starting the counter from 1 instead of the default value of 0. This is done by passing the value 1 as the second argument to the enumerate() function.
Example 3: Using the enumerate() function with a string
“`
string = “Hello, World!”
for i, letter in enumerate(string):
print(i, letter)
“`
Output:
“`
0 H
1 e
2 l
3 l
4 o
5 ,
6
7 W
8 o
9 r
10 l
11 d
12 !
“`
In this example, the enumerate() function is applied to a string. The function loops through each character of the string and generates tuples with an index value and the corresponding character.
Conclusion
In conclusion, the enumerate() function is a powerful built-in function in Python that simplifies coding by iterating through a sequence while at the same time keeping track of the element index. This function can be used to loop over any iterable object such as lists, tuples, and strings. With the examples given in this article, you now know how to use the Python enumerate() function in your own code.