How to Connect Your React App to Firebase
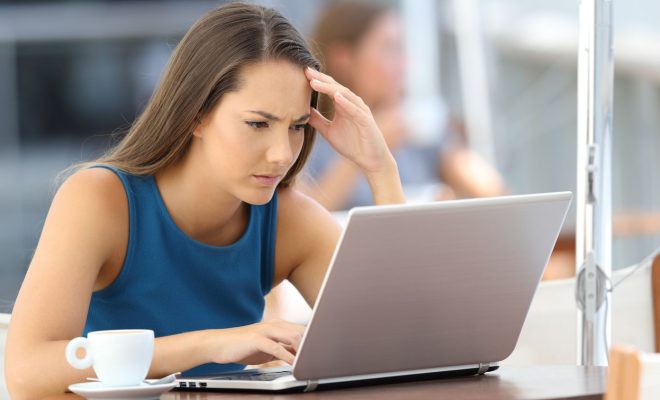
Firebase is a cloud-based platform that provides effective tools to develop mobile and web applications. It offers several features like authentication, real-time database, storage and many more. One of the most significant advantages of Firebase is its ability to connect with various front-end frameworks such as React. In this article, we will discuss how to connect your React app to Firebase using the Firebase JavaScript SDK.
Step 1: Create a Firebase Project and Set Up Firestore
the first step to connecting your React app to Firebase is to create a Firebase project. Go to the Firebase website and create an account if you don’t have one. After logging in, click on the “Create a Project” button and provide a suitable name and description for your project.
Once the project is created, click on the “Get started” button to set up Firebase Firestore, a cloud-based NoSQL document database. Firestore can help you store and manage application data efficiently. Follow the on-screen instructions to enable Firestore for your project.
Step 2: Install the Firebase JavaScript SDK in your React-app
the next step is to install the Firebase JavaScript SDK in your React project. Open the terminal of your project and run the following commands:
“
npm install firebase
“
This command will install the Firebase SDK and its dependencies.
Step 3: Initialize Firebase in Your React App
The next step is to initialize Firebase in your React App by setting up the configuration parameters. To get them, go to your Firebase console and select your project. Click on the “Project Overview,” then click on the “Add Firebase to your web app” option. Copy the code snippet from there and paste it into your React app’s main JavaScript file, typically ‘index.js.’
Before running this code, replace the API keys and other initialization parameters with your Firebase project’s values.
“
// Import the Firebase Core module
import firebase from ‘firebase/app’;
// Import the Firebase Database module
import ‘firebase/firestore’;
// Replace the values with your Firebase project’s values
const firebaseConfig = {
apiKey: ‘YOUR_API_KEY’,
authDomain: ‘YOUR_AUTH_DOMAIN’,
projectId: ‘YOUR_PROJECT_ID’,
storageBucket: ‘YOUR_STORAGE_BUCKET’,
messagingSenderId: ‘YOUR_MESSAGING_SENDER_ID’,
appId: ‘YOUR_APP_ID’,
measurementId: ‘YOUR_MEASUREMENT_ID’
};
// Initialize Firebase app instance
firebase.initializeApp(firebaseConfig);
// Initialize Firestore
const firestore = firebase.firestore();
“
Step 4: Perform CRUD Operations in Firestore
After initializing Firestore, you can perform the CRUD (Create, Read, Update, Delete) operations using the Firestore API. To perform CRUD operations in Firestore, you must define the Database schema or data model first.
Here’s how to define a simple data model in a React app:
“
class userCollection {
constructor() {
this.users = firestore.collection(‘users’);
}
async getAllUsers() {
const snapshot = await this.users.get();
return snapshot.docs.map(doc => doc.data());
}
async addUser(user) {
const newUser = await this.users.add(user);
return newUser.id;
}
async updateUser(id, user) {
await this.users.doc(id).update(user);
return id;
}
async deleteUser(id) {
await this.users.doc(id).delete();
return id;
}
}
“
The code above defines a User Collection, which has the following CRUD methods: getAllUsers, addUser, updateUser, and deleteUser.
Conclusion
by following the above-provided steps, you can quickly connect your React App to Firebase and start integrating amazing features into your application with Firestore. Firebase has many other features such as authentication, storage, and others that can be useful for your React application. Go ahead and explore Firebase for amazing features that you can integrate into your React app.