Different Ways of Writing Conditional Statements in JavaScript
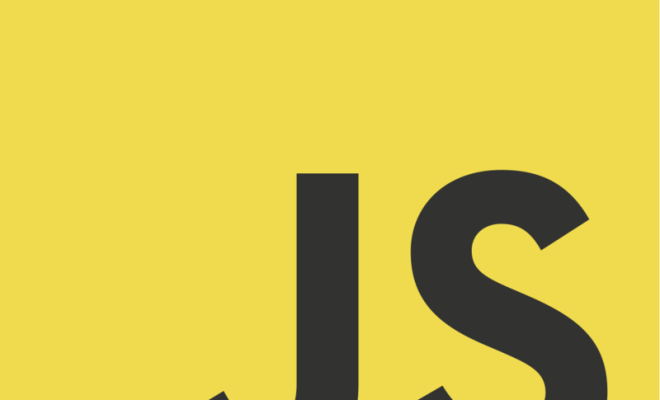
Conditional statements are an essential aspect of programming regardless of the language used. In JavaScript, conditionals help you control the flow of your program based on logic. They are used to execute different statements based on whether a condition is true or false. There are different ways to write conditional statements in JavaScript, each with its own advantages and disadvantages.
- If Statement
The if statement is one of the most commonly used conditional statements in JavaScript. It allows you to execute a block of code if a condition is true. If the condition is false, the block of code is skipped, and the program continues with the next statement.
Here is an example:
if (score >= 60) {
console.log(“You have passed the exam!”);
}
- If-Else Statement
The if-else statement is used when you want to execute a different statement when the condition is false. The else block is executed whenever the condition in the if statement is false.
Here is an example:
if (score >= 60) {
console.log(“You have passed the exam!”);
} else {
console.log(“You have failed the exam!”);
}
- Else-If Statement
An else-if statement allows you to test multiple conditions and execute different blocks of code based on them.
Here is an example:
if (score >= 90) {
console.log(“You have received an A!”);
} else if (score >=80) {
console.log(“You have received a B!”);
} else if (score >= 70) {
console.log(“You have received a C!”);
} else {
console.log(“You have failed the exam!”);
}
- Ternary Operator
The ternary operator is a shorthand way of writing an if-else statement in a single line of code.
Here is an example:
var result = (score >= 60) ? “You have passed the exam!” : “You have failed the exam!”;
console.log(result);
- Switch Statement
The switch statement is used when you have multiple conditions to compare against. It allows you to test a variable against a list of cases and execute different blocks of code based on the matching case.
Here is an example:
switch(day) {
case “Monday”:
console.log(“Today is Monday!”);
break;
case “Tuesday”:
console.log(“Today is Tuesday!”);
break;
default:
console.log(“Today is not Monday or Tuesday.”);
}