What Is the Diamond Problem in C++? How to Spot It and How to Fix It
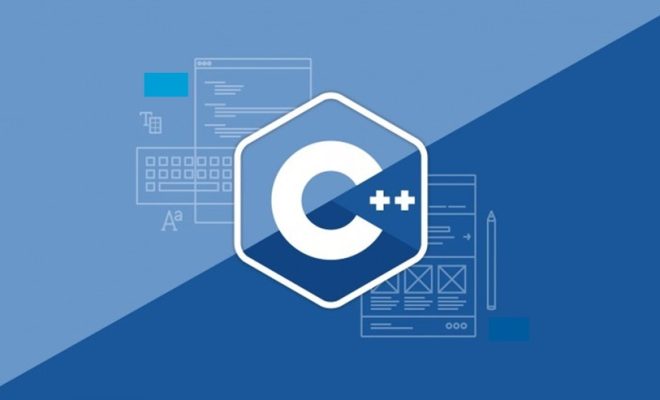
C++ is a popular programming language used for a variety of applications. However, like any programming language, it has its quirks and challenges. One such challenge is known as the diamond problem, which occurs when multiple inheritance is used in a class hierarchy. This article will explore what the diamond problem is, how to spot it, and how to fix it.
What Is the Diamond Problem?
The diamond problem occurs in C++ when two classes inherit from a common base class, and a third class inherits from both of these classes. The resulting class hierarchy resembles a diamond shape, with the common base class at the top of the diamond and the two derived classes at the bottom corners. This makes it difficult for the third class, which inherits from both derived classes, to know which version of the inherited method to use. This can result in conflicts and ambiguity in the program.
How to Spot the Diamond Problem
To spot the diamond problem, look for a class hierarchy that resembles a diamond shape, as described above. If there are multiple derived classes that inherit from a common base class and a third class that inherits from both of these derived classes, the diamond problem is likely to occur.
Additionally, if there are conflicting methods or variables in the derived classes, this can also indicate the diamond problem. The third class that inherits from both derived classes may not know which version of the method to use, leading to conflicts and ambiguity in the program.
How to Fix the Diamond Problem
There are several ways to fix the diamond problem in C++. One solution is to use virtual inheritance, which ensures that only one instance of the base class is created in the derived class hierarchy. This eliminates the ambiguity and conflicts that arise in the diamond problem.
Virtual inheritance is implemented by using the keyword “virtual” when inheriting from the common base class. This tells the compiler to create a single instance of the base class in the derived class hierarchy. The syntax for virtual inheritance looks like this:
class Base {
// …
};
class Derived1 : virtual public Base {
// …
};
class Derived2 : virtual public Base {
// …
};
class Third : public Derived1, public Derived2 {
// …
};
In this example, both Derived1 and Derived2 inherit virtually from the Base class, and the Third class inherits from both Derived1 and Derived2.
Another solution is to use composition instead of inheritance. This involves creating an object of the base class inside the derived class, rather than inheriting from it. This reduces the complexity of the class hierarchy and eliminates the diamond problem.