Understanding Constructors in JavaScript
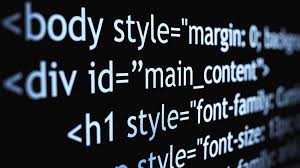
JavaScript is one of the most popular programming languages in the world, and it is used by millions of developers to create incredible web and mobile applications. One of the key features of JavaScript is the constructor function, which allows developers to create new objects easily and efficiently.
Understanding constructors in JavaScript is important for any developer who wants to create complex and interactive web applications. In this article, we will discuss constructors in detail and explain how they can be used to create new objects.
What is a constructor in JavaScript?
A constructor is a special function in JavaScript that is used to create new objects. It is called so because the function is used to ‘construct’ or create a new object. When a new object is created using a constructor function, it is called an instance of that constructor.
In JavaScript, constructors are used to define the blueprint of an object. The blueprint defines the properties and methods that the object will have. When a new instance of the object is created using the constructor function, it inherits all the properties and methods defined in the blueprint.
How to create a constructor in JavaScript?
Creating a constructor in JavaScript is very easy. All you need to do is define a function that represents the constructor and add properties and methods to it. Here is an example of how to create a constructor in JavaScript:
“`javascript
function Person(name, age) {
this.name = name;
this.age = age;
}
let person1 = new Person(“John”, 25);
let person2 = new Person(“Jane”, 30);
“`
In the above example, we define a constructor function called `Person`. The function takes two parameters, `name` and `age`, which are used to set the `name` and `age` properties of the object.
To create a new instance of the `Person` object, we use the `new` keyword followed by the constructor function name and pass in the parameters required by the constructor.
Why use constructors in JavaScript?
Constructors are used in JavaScript for many reasons. Here are a few of the most important ones:
1. Creating multiple instances of an object: Constructors allow you to create multiple instances of the same object with different values for its properties.
2. Encapsulation: Constructors can be used to encapsulate properties and methods that relate to a specific object. This ensures that the properties and methods are available only to the object and not to other parts of the code.
3. Inheritance: Constructors can also be used to create object hierarchies, where one object inherits properties and methods from another object. This helps to create more complex and organized code that is easier to maintain.
Conclusion
Constructors are an important feature of JavaScript that allows developers to create new objects easily and efficiently. They are used to define the blueprint of an object and can be used to create multiple instances of an object with different values for its properties. Understanding constructors in JavaScript is important for any developer who wants to create complex and interactive web applications.