How to Style React Components Using CSS Modules
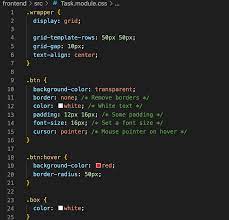
React is a powerful JavaScript library that allows developers to build robust and dynamic web applications. With its components-based architecture, React makes it easy for developers to create reusable and modular UI elements. But when it comes to styling these components, things get a bit complicated.
Traditionally, styling for React components has been done using CSS or SASS. But with the introduction of CSS Modules, a new approach has emerged. CSS Modules provide developers with an efficient way to style React components while avoiding the issues associated with traditional CSS.
In this article, we will explore how to style React components using CSS Modules.
What are CSS Modules?
CSS Modules are a way of writing modular CSS that eliminates the issues associated with traditional CSS. With CSS Modules, each CSS file is treated as a separate module, and every class within that module is scoped to that module. This means that classes are not globally available, which avoids the issue of styles bleeding over into other components.
CSS Modules work by generating a unique class name for every element in your component. This unique class name is created by combining the class name with a unique hash. The output is a class name that is unique to your component and will not conflict with other components in your application.
How to Use CSS Modules
To use CSS Modules in a React project, you will need to install a loader for your CSS files. There are several loaders available, but we will be using the CSS Loader.
1. Install the CSS Loader
The first step is to install the CSS Loader. You can do this by running the following command in your terminal window:
“`
npm install css-loader –save-dev
“`
2. Configure your webpack.config.js file
Next, you will need to configure your webpack.config.js file to use the CSS Loader. Add the following code to your webpack.config.js file:
“`
{
test: /\.css$/,
use: [‘style-loader’, ‘css-loader’]
}
“`
This will tell webpack to use the CSS Loader when it encounters a .css file.
3. Import your CSS files
Now that you have installed the CSS Loader and configured your webpack.config.js file, you can start using CSS Modules in your React components.
To import your CSS files, you can use the import statement in your component file like so:
“`
import styles from ‘./styles.module.css’;
“`
This will import your CSS file as a JavaScript object, with each class name mapping to a unique identifier.
4. Use your CSS Modules classes
Finally, you can use your CSS Modules classes in your React components. You can access your classes by using the object that was created when you imported your CSS file. To apply a class to an element, simply add the class name to the element’s className attribute like so:
“`
Hello World
“`
Conclusion
CSS Modules provide a powerful way to style React components while avoiding the issues associated with traditional CSS. By generating unique class names for each component, CSS Modules eliminate the risk of styles bleeding over into other components. By following the steps outlined in this article, you can start using CSS Modules in your own React projects and take advantage of their modularity and efficiency.