How to Find the Sum of All Elements in an Array
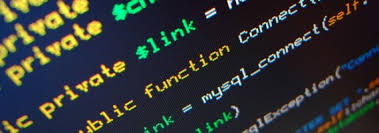
Arrays are a popular data structure used in programming for storing multiple values of the same data type. Often times, when working with arrays, we may want to find the sum of all the elements in the array. This can be accomplished easily using a for-loop and a variable to keep track of the sum.
Here is a step-by-step guide on how to find the sum of all elements in an array:
Step 1: Declare an array
The first step is to declare and initialize an array of integers. For example, we can declare an array of 5 integer values as follows:
int[] arr = {3, 5, 2, 8, 1};
Step 2: Set a variable to zero
Next, we will set a variable to zero which will be used to store the sum of all the elements in the array. We can do this as follows:
int sum = 0;
Step 3: Use a for-loop to iterate over the array
Now, using a for-loop, we can iterate over the array and add up all the elements to our sum variable. We start from index 0 and continue until the last index of the array which can be obtained using the length property of the array. The for-loop looks like this:
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
Step 4: Print the sum value
Finally, we can print out the sum of all the elements in the array. This can be done using the System.out.println() method as follows:
System.out.println(“Sum of all elements in the array: ” + sum);
The complete code for finding the sum of all elements in an array is given below:
public class Main {
public static void main(String[] args) {
int[] arr = {3, 5, 2, 8, 1};
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
System.out.println(“Sum of all elements in the array: ” + sum);
}
}
Output:
Sum of all elements in the array: 19