How to Create a Protected Route in React
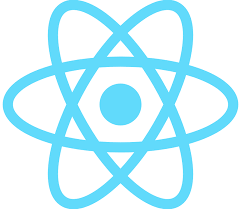
React is a popular JavaScript library used for building user interfaces. It is robust and efficient, making it a favored choice among developers. In this article, we will learn how to create a protected route in React.
A protected route is a route that requires authentication or authorization before accessing it. It is useful in applications that require different levels of access based on user roles. For example, a user may be allowed to access a dashboard only after logging in. Creating a protected route in React involves the following steps.
1. Install Dependencies
You need to install React Router and React Router DOM to create protected routes. These modules allow you to define routes in your application and handle navigation between them easily.
To install these dependencies, run the following command in your terminal:
“`
npm install react-router-dom
“`
2. Define Routes
Next, you need to define the routes in your application. React Router provides a Route component that you can use to define these routes. Each Route component takes two props: path and component. The path prop specifies the URL path, while the component prop specifies the corresponding component to render.
For example, let’s say we have two components: Dashboard and Home. We can define the routes like this:
“`
import { Route } from ‘react-router-dom’;
import Dashboard from ‘./Dashboard’;
import Home from ‘./Home’;
function App() {
return (
);
}
export default App;
“`
In this example, the Home component will be rendered when the user visits the root path, while the Dashboard component will be rendered when the user visits the /dashboard path.
3. Create a Private Route Component
To create a protected route, we need to create a new component that extends the Route component and adds some logic to check whether the user is authenticated. If the user is not authenticated, the component should redirect to a login page.
Here’s an example of a PrivateRoute component:
“`
import React from ‘react’;
import { Route, Redirect } from ‘react-router-dom’;
const PrivateRoute = ({ component: Component, isAuthenticated, …rest }) => {
return (
<route
</route
{…rest}
render={(props) =>
isAuthenticated ? (
) : (
)
}
/>
);
};
export default PrivateRoute;
“`
In this example, the PrivateRoute component takes three props: component, isAuthenticated, and rest. The component prop specifies the component to be rendered when the user is authenticated. The isAuthenticated prop is a boolean value that indicates whether the user is authenticated. The rest prop contains any other props that might be passed to the Route component.
The PrivateRoute component uses the render prop of the Route component to check whether the user is authenticated. If the user is authenticated, it renders the specified component. If not, it redirects the user to the login page.
4. Use PrivateRoute in the App Component
Finally, we need to use the PrivateRoute component in the App component instead of the Route component. We also need to pass the isAuthenticated prop to the PrivateRoute component.
Here’s an example of how to use the PrivateRoute component:
“`
import React, { useState } from ‘react’;
import { BrowserRouter as Router } from ‘react-router-dom’;
import PrivateRoute from ‘./PrivateRoute’;
import Dashboard from ‘./Dashboard’;
import Login from ‘./Login’;
function App() {
const [isAuthenticated, setIsAuthenticated] = useState(false);
const handleLogin = () => {
setIsAuthenticated(true);
};
const handleLogout = () => {
setIsAuthenticated(false);
};
return (
<privateroute
</privateroute
exact
path=”/dashboard”
component={Dashboard}
isAuthenticated={isAuthenticated}
/>
<route
</route
exact
path=”/login”
render={(props) => (
)}
/>
);
}
export default App;
“`
In this example, we use the useState hook to create a boolean state variable called isAuthenticated that indicates whether the user is authenticated. We also define two functions handleLogin and handleLogout that update the isAuthenticated state.
In the App component, we render two routes: a PrivateRoute for the Dashboard component and a regular Route for the Login component. We pass the isAuthenticated prop to the PrivateRoute component, which is used to determine whether the Dashboard component should be rendered.
Conclusion
Creating a protected route in React is an essential skill for building secure applications. React Router makes it easy to define routes in your application, and the PrivateRoute component allows you to implement authentication and authorization easily. By following the steps outlined in this article, you can create a protected route in React and keep your application secure.