How to Build Drag and Drop Components in React
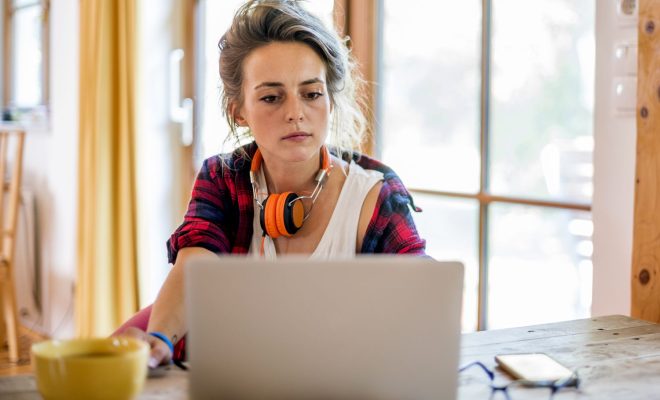
React is a popular JavaScript library used for building user interfaces. One of the popular features of React is its ability to create drag and drop components. In this article, we will learn how to build drag and drop components in React.
Before we start, let’s understand what React is. React is a JavaScript library that allows us to build user interfaces with reusable components, making the code more organized, easier to maintain, and simpler to debug.
Drag and drop functionality is an important feature of modern web applications. It allows users to easily move items around on a web page, making it more interactive and user-friendly. With the help of React, we can easily implement drag and drop functionality in our web applications.
Let’s take a look at the steps to build a drag and drop component in React.
Step 1 – Install React Create App
React Create App allows us to create a new React project with just one command-line tool. To install React Create App, run the following command in the terminal:
“`
npx create-react-app my-app
“`
Here, *my-app* is the name of our application.
Step 2 – Install React Drag and Drop Library
To build drag and drop components, we need to install the React Drag and Drop Library. Run the following command in the terminal:
“`
npm install –save react-dnd react-dnd-html5-backend
“`
Here, *npm* is the package manager for JavaScript, and *–save* specifies that we want to save the package as a dependency in our project.
Step 3 – Create a New Component
In the *src* folder, create a new component. Name the component *DragAndDrop*.
“`
import React from ‘react’;
const DragAndDrop = () => {
return (
Drag and Drop Example
);
}
export default DragAndDrop;
“`
Step 4 – Add Drag and Drop Functionality
To add drag and drop functionality to our component, we need to use the *useDrop* and *useDrag* hooks from the React Drag and Drop Library. The *useDrop* hook allows us to specify where items can be dropped, and the *useDrag* hook allows us to specify the item that can be dragged.
“`
import React from ‘react’;
import { useDrop, useDrag } from ‘react-dnd’;
import ‘./DragAndDrop.css’;
const DragAndDrop = () => {
const [{ isDragging }, drag] = useDrag(() => ({
type: ‘card’,
collect: (monitor) => ({
isDragging: monitor.isDragging(),
}),
}));
const [{ canDrop, isOver }, drop] = useDrop({
accept: ‘card’,
collect: (monitor) => ({
isOver: monitor.isOver(),
canDrop: monitor.canDrop(),
}),
});
const getBackgroundColor = () => {
if (canDrop) {
return ‘#f7f7f7’;
}
return ‘#ffffff’;
};
return (
<div
</div
ref={drop}
style={{ backgroundColor: getBackgroundColor() }}
className=’card’
>
<div
</div
ref={drag}
style={{ opacity: isDragging ? 0.5 : 1 }}
className=’card-body’
>
Card Title
Card Description
);
};
export default DragAndDrop;
“`
Step 5 – Style the Component
Finally, we need to style our component. Here’s an example of how to style the component with CSS.
“`
.card {
width: 300px;
margin: 20px;
border: 2px solid black;
border-radius: 5px;
}
.card-body {
padding: 10px;
cursor: move;
}
.card-title {
font-size: 18px;
margin: 0;
}
.card-text {
font-size: 14px;
margin: 0;
color: #8f8f8f;
}
“`
Step 6 – Add to Main Component
To see the final result, we need to add the *DragAndDrop* component to our main component. Here’s an example of how to do that.
“`
import React from ‘react’;
import DragAndDrop from ‘./components/DragAndDrop/DragAndDrop’;
const App = () => {
return (
);
};
export default App;
“`
Congratulations! Now we know how to build drag and drop components in React. With this knowledge, we can enhance our web applications and make them more interactive and user-friendly.