How to Batch Rename Files in Python
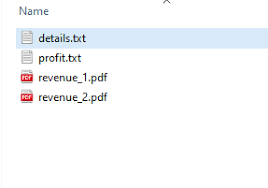
Batch renaming files can be a tedious and time-consuming task, especially when you have a large number of files to rename. This is where Python comes in – it offers a powerful and flexible way to automate this process, saving you time and effort.
In this article, we will explore how to batch rename files in Python.
Step 1: Importing the necessary modules
To begin with, we need to import the necessary modules that will help us rename the files. The ‘os’ module is one such module that is required as it provides the required functionality to interact with the file system.
“`
import os
“`
Step 2: Setting the directory path
Once we have imported the ‘os’ module, we need to set the directory path where the files to be renamed are located. We can do this by using the ‘os.chdir()’ function.
“`
os.chdir(‘/path/to/folder’)
“`
Step 3: Listing the files in the directory
Next, we need to list all the files that are present in the directory. We can use the ‘os.listdir()’ function to achieve this.
“`
files = os.listdir(‘.’)
“`
Here, the ‘.’ represents the current directory. If you want to specify a different directory, you can mention the path relative to the directory where the Python script is located.
Step 4: Renaming the files
Now that we have the list of files that need to be renamed, we can proceed to rename them. We will use a ‘for’ loop to iterate through the list of files and rename them one by one.
“`
for file_name in files:
new_file_name = ‘new_file_name_’ + file_name
os.rename(file_name, new_file_name)
“`
In the above code, we have concatenated the string ‘new_file_name_’ with the original file name to create a new file name. You can change this to suit your requirements.
Step 5: Complete code
Putting all the above steps together, we get the following code:
“`
import os
os.chdir(‘/path/to/folder’)
files = os.listdir(‘.’)
for file_name in files:
new_file_name = ‘new_file_name_’ + file_name
os.rename(file_name, new_file_name)
“`
This code should work for most use cases of batch renaming files. However, it’s always a good idea to test the code on a few sample files before running it on a large number of files.
Conclusion
Batch renaming files in Python is a powerful way to automate a tedious process. By using the steps outlined in this article, you should be able to easily rename multiple files with minimal effort. So, go ahead and try it out for yourself!