Create a Progress Bar in Python CLI
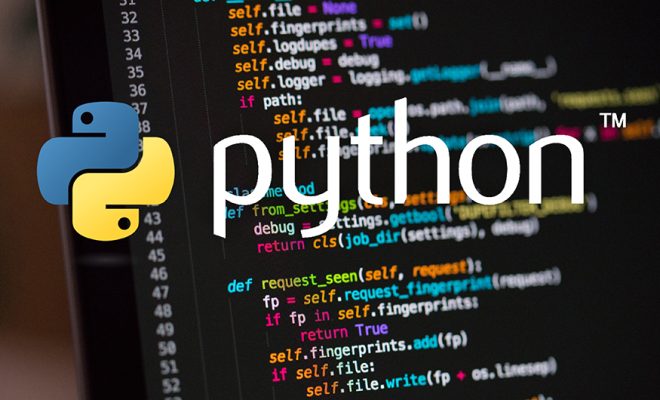
As a Python developer, you may need to display results of your scripts on a console. One way to enhance the user experience is to display a progress bar, especially if our script takes long to run. In this article, we will learn how to create a progress bar in Python CLI.
First, let us look at a simplistic way of displaying a progress bar. Consider the following script that takes a long time to run:
“`
import time
for i in range(0, 101):
time.sleep(0.1)
print(i)
“`
The script simply counts from 0 to 100 and waits for 0.1 seconds before printing the next count. While this is running, let us assume that we want to display the progress of the script in the following format: `[####——–] 40%`
To achieve this, we need the following:
1. A counter that keeps track of the progress of our script
2. A string representation of the progress bar that we can update as the script runs.
3. A way of printing the progress bar and updating it as the script runs.
Let’s implement the above-mentioned requirements:
“`
import time
def progress(count, total, status=”):
bar_len = 60
filled_len = int(round(bar_len * count / float(total)))
percents = round(100.0 * count / float(total), 1)
bar = ‘#’ * filled_len + ‘-‘ * (bar_len – filled_len)
print(‘[%s] %s%s %s\r’ % (bar, percents, ‘%’, status), end=”)
total = 100
for i in range(1, total + 1):
time.sleep(0.1)
progress(i, total)
“`
The `progress` function takes in three arguments:
1. `count`: the current count of the script
2. `total`: the total number of iterations
3. `status`: any additional messages you want to display while the script runs.
The function calculates the percentage completed by dividing `count` by `total`. It then creates a string representation of the progress bar using the `bar` and `filled_len` variables.
The output of the function is then printed to the console, with the `\r` character included to ensure that the output overwrites any previous output.
Finally, we call the `progress` function within the for loop, passing in the `i` and `total` values to calculate progress.
We have effectively implemented a progress bar in Python CLI. You can run this script on any long-running script to enhance the user experience.
In conclusion, the progress bar is an essential tool for enhancing the user experience when running long scripts. By displaying the progress of the script in a visual way, users can track the progress of a script. In Python, creating the progress bar is straightforward, and with the implementation we have discussed in this article, Python developers can easily add progress bars to their CLI-based applications.