Timer Mechanisms With C and Linux
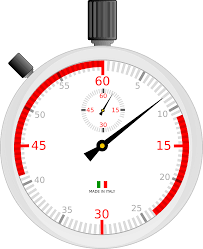
Timer mechanisms are an essential aspect of any software development that requires accurate timing. They enable developers to schedule events, measure and track time-sensitive tasks, and execute tasks at specific intervals. One of the most popular programming languages for building timer mechanisms is C, a general-purpose, imperative computer programming language that is widely used in operating systems, embedded systems, and microcontrollers. In this article, we will explore how to implement timer mechanisms using C programming language in Linux and learn about the benefits of using C for timer mechanism development.
Timer Mechanisms in C
A timer mechanism consists of two essential components: a timer source and a timer handler. C provides two types of timers: software timers and hardware timers. Software timers are implemented using software processes and are usually used for short-term timing tasks, such as event handling or signal delivery. On the other hand, hardware timers are implemented in the hardware of the system and are used for long-term timing tasks, such as measuring performance or monitoring system states.
Software Timer Mechanism with C
In C programming, a software timer mechanism can be developed using signal handling. Signals are software interrupts that can be sent to a process to notify it of an event. C programming provides a signal library named “signal.h” to handle signals. The timer function is implemented using the alarm function provided by the signal.h library. The alarm function takes an argument in seconds and starts a timer. Once the timer expires, it sends a signal (SIGALRM) to the process.
Here is an example of how to implement a software timer mechanism in C:
#include
#include
#include
void timer_handler(int sig)
{
printf(“Timer expired\n”);
exit(0);
}
int main()
{
signal(SIGALRM, timer_handler);
alarm(5);
while(1){};
return 0;
}
The above code sets up a signal handler that will print “Timer expired” once the timer expires. The timer will expire after 5 seconds, and the program will exit.
Hardware Timer Mechanism with C
In C programming language, a hardware timer mechanism can be developed using timers provided by the operating system or microcontroller firmware. In Linux, the timer function is implemented using the kernel timer interrupts. The timer interrupt is a hardware interrupt that is generated by a hardware timer. The kernel schedules the timer interrupt at a specific time, and when the timer interrupt occurs, the kernel executes the timer handler. The timer handler is executed in the kernel context and can perform any required operations at that time.
Here is an example of how to implement a hardware timer mechanism in C:
#include
#include
#include
volatile sig_atomic_t flag = 0;
void timer_handler(int sig)
{
flag = 1;
}
int main()
{
struct itimerval timer;
signal(SIGALRM, timer_handler);
timer.it_value.tv_sec = 2;
timer.it_value.tv_usec = 0;
timer.it_interval.tv_sec = 2;
timer.it_interval.tv_usec = 0;
setitimer(ITIMER_REAL, &timer, NULL);
while(1)
{
if(flag == 1)
{
printf(“Timer expired\n”);
flag = 0;
}
}
return 0;
}
The above code sets up a timer interrupt that will occur every 2 seconds. Once the timer interrupt occurs, the timer handler will print “Timer expired” and notify the flag variable.
Benefits of C for Timer Mechanism Development
C programming language is widely used in operating systems, embedded systems, and microcontroller platforms due to its lightweight nature and efficient memory management. Despite the increasing popularity of high-level programming languages like Python, C is still the most popular language for system-level programming, and timer mechanism development is no exception. Here are some benefits of using C for timer mechanism development:
1. Lightweight – C language is a lightweight programming language and is suitable for use in resource-constrained environments, such as microcontrollers.
2. Efficient Memory Management – C language provides control over memory management, which means a programmer can choose when to allocate and deallocate memory, reducing unnecessary memory usage.
3. Accessibility – C language is a widely known language and has a vast amount of resources available for learning and development, making it more accessible for developers.
Conclusion
In summary, C programming language is an excellent choice for building timer mechanisms in Linux operating systems or microcontroller systems. The lightweight nature and efficient memory management make C a suitable language for use in resource-constrained environments, while the built-in signal library and hardware timers make it perfect for accurate and precise time scheduling. By building your timer mechanisms with C, you can take advantage of its performance and industry-standard programming methods, making your timer mechanisms efficient, reliable, and accessible to other developers.