How to Zip and Unzip Files Using Python
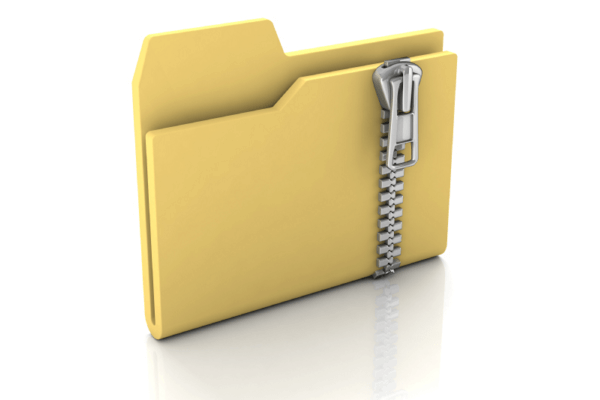
Zipping and unzipping files is a common task in software development. Python provides built-in modules to handle this task easily. In this article, we will learn how to zip and unzip files using Python.
Zipping Files
To zip a file, we can use the shutil module in Python. Let’s consider a scenario where we want to compress two files – “file1.txt” and “file2.txt” – into a zip file “compressed_files.zip”. Here is the code:
“`
import shutil
file_paths = [“file1.txt”, “file2.txt”]
zip_path = “compressed_files.zip”
with zipfile.ZipFile(zip_path, “w”) as zip_obj:
for file in file_paths:
zip_obj.write(file)
“`
In this code, we first import the shutil module. Then we define the list of file paths that we want to zip and the destination zip file path. The next step is to open the zip file object in write mode. We use a with statement to ensure that the zip_obj is properly closed when the code block is finished executing.
Next, we loop through the list of file paths and use the write() method of the zip_obj to write each file to the zip file. The write() method takes the file path as an argument.
Finally, we close the zip file object. Now, when we run this code, the two files “file1.txt” and “file2.txt” will be compressed into the “compressed_files.zip” file.
Unzipping Files
To unzip a file, we can again use the shutil module. Here is the code:
“`
import shutil
zip_path = “compressed_files.zip”
extract_path = “unzipped_files”
with zipfile.ZipFile(zip_path, “r”) as zip_obj:
zip_obj.extractall(extract_path)
“`
In this code, we first define the zip file path and the path where we want to extract the files. We open the zip file object in read mode using the with statement.
We then use the extractall() method of the zip_obj to extract all files to the specified extract_path.
Now that we have unzipped the files, we can work with them as we would with any other files.
Conclusion
Zipping and unzipping files using Python is a simple task that can be performed using the built-in modules. The shutil module provides a convenient way to compress and extract files. By using the code examples above, you can easily zip and unzip files in your Python projects.