How to Read Strings From a .env File With Python, Express.JS, and Go
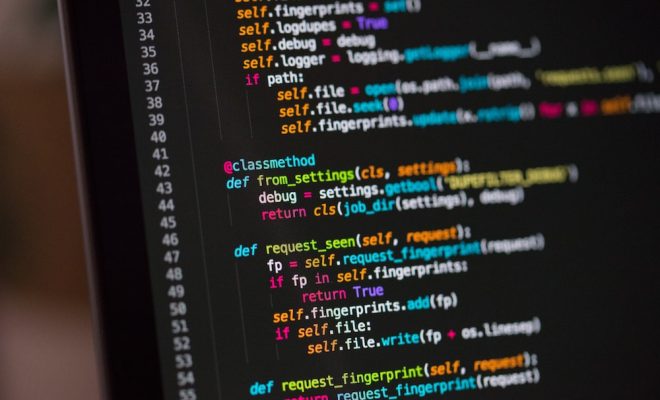
If you’re building a web application, chances are you don’t want to hardcode important pieces of information like API keys, database credentials, or other secrets directly into your source code. Instead, you can store these values in a special configuration file called a .env file.
In this article, we’ll cover how to read strings from a .env file using Python, Express.JS (a popular web framework for Node.js), and Go.
Python
Python is a popular language for web development, and there are several libraries available that make it easy to read values from a .env file. One such library is python-dotenv.
To use python-dotenv, first install it using pip:
“`pip install python-dotenv“`
Then, create a .env file in your project directory with your key-value pairs:
“`
API_KEY=12345
DATABASE_URL=postgres://user:password@localhost/mydatabase
“`
Now, in your Python script, import the dotenv library and load the .env file:
“`python
import os
from dotenv import load_dotenv
load_dotenv()
api_key = os.getenv(“API_KEY”)
database_url = os.getenv(“DATABASE_URL”)
“`
Note that we call the `load_dotenv()` function before attempting to access any values. This ensures that all the key-value pairs in the .env file are loaded into environment variables, which can be accessed using the `os.getenv()` function.
Express.JS
Express.JS is a popular web framework for Node.js, and it also has several libraries available that make it easy to read values from a .env file. One such library is dotenv.
To use dotenv, first install it using npm:
“`npm install dotenv“`
Then, create a .env file in your project directory with your key-value pairs:
“`
API_KEY=12345
DATABASE_URL=postgres://user:password@localhost/mydatabase
“`
Now, in your main Node.js application file, load the .env file using dotenv:
“`javascript
require(‘dotenv’).config()
const apiKey = process.env.API_KEY
const databaseUrl = process.env.DATABASE_URL
“`
Note that we call `require(‘dotenv’).config()` at the beginning of our file to load the .env file into environment variables. We can then access these variables using `process.env`.
Go
Go is another popular language for web development, and it also has built-in support for reading values from environment variables. However, there is a popular third-party library called godotenv that makes it easier to load values from a .env file.
To use godotenv, first install it using go get:
“`go get github.com/joho/godotenv“`
Then, create a .env file in your project directory with your key-value pairs:
“`
API_KEY=12345
DATABASE_URL=postgres://user:password@localhost/mydatabase
“`
Now, in your main Go file, load the .env file using godotenv:
“`go
import (
“github.com/joho/godotenv”
“os”
)
func main() {
godotenv.Load()
apiKey := os.Getenv(“API_KEY”)
databaseUrl := os.Getenv(“DATABASE_URL”)
}
“`
Note that we call `godotenv.Load()` at the beginning of our file to load the .env file into environment variables. We can then access these variables using `os.Getenv()`.
Conclusion
Reading values from a .env file can greatly simplify the management of sensitive information in your web application. Whether you’re using Python, Express.JS, Go, or any other language, there are libraries available that make it easy to load these values into environment variables. Happy coding!