How to Draw Different Shapes Using a Turtle in Python
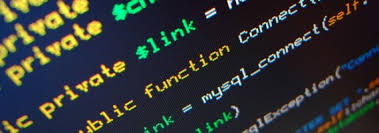
Python is a widely-used programming language that is widely known for being beginner-friendly, easy to learn, and very flexible. One of its many strengths is its ability to be used for graphics programming, including drawing complex shapes and images. One of the most popular Python libraries for graphics programming is the turtle module. In this article, we will explore how to use the turtle module in Python to draw different shapes.
Before we begin, make sure that you have Python and the turtle module installed on your computer. You can download and install Python from the official website, https://www.python.org/downloads/, while the turtle module is included in Python’s standard library.
The turtle module is based on the concept of a turtle moving around a canvas, leaving behind a trail as it moves. To begin, we must first import the turtle module:
“`
import turtle
“`
This statement will allow us to use the turtle module in our Python script.
Next, we must create a turtle object. We can do this by calling the Turtle() function provided by the turtle module:
“`
my_turtle = turtle.Turtle()
“`
Now that we have a turtle object, we can start drawing shapes. Let’s start with the most basic shape, a line.
To draw a line, we can use the forward() method of the turtle object. This method moves the turtle forward by a specified distance, leaving behind a trail as it moves. Here’s an example:
“`
my_turtle.forward(100)
“`
This statement will move the turtle forward by 100 units. The unit of measurement depends on the scale of your canvas.
To draw a triangle, we can use the forward() method to move the turtle forward by a certain distance, and then use the left() or right() method to change the turtle’s direction. Here’s an example:
“`
my_turtle.forward(100)
my_turtle.left(120)
my_turtle.forward(100)
my_turtle.left(120)
my_turtle.forward(100)
“`
This code will draw a triangle with each side measuring 100 units.
To draw a rectangle, we can use the forward() method to move the turtle forward and the left() or right() method to change the turtle’s direction at each corner. Here’s an example:
“`
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(50)
my_turtle.left(90)
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(50)
“`
This code will draw a rectangle with sides of length 100 and 50 units.
To draw a circle, we can use the circle() method of the turtle object. This method takes a radius as its argument and draws a circle with that radius. Here’s an example:
“`
my_turtle.circle(50)
“`
This code will draw a circle with a radius of 50 units.
To draw a square, we can combine the techniques of drawing a rectangle and a triangle. Here’s an example:
“`
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(100)
“`
This code will draw a square with sides of length 100 units.
In conclusion, the turtle module in Python is a powerful tool for graphics programming. With just a few simple commands, we can draw a wide variety of shapes and objects. With some practice, we can create even more complex designs and animations using the turtle module.