Bash Variables Explained: A Simple Guide with Examples
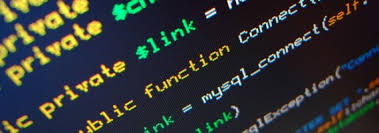
Bash, short for Bourne-Again SHell, is a Unix shell and a command-line interpreter that is widely used on Linux and other Unix-like operating systems. In Bash, variables are containers that hold data, such as text strings, numbers, or arrays. They are used to store and manipulate data, and are essential in writing scripts and automating tasks.
In this article, we will go over the different types of Bash variables and explain how to declare and use them. We will also provide some examples to illustrate their use.
Types of Bash Variables
Bash supports several types of variables, including:
1. Local Variables: These are variables that are defined and used in a script or function. They have limited scope and are not accessible outside of the script or function.
2. Environment Variables: These are variables that are set and used by the operating system or other programs. They have global scope and are accessible by all processes.
3. Shell Variables: These are variables that are set and used by the shell itself. They have intermediate scope and are accessible by all processes that are launched from the shell.
Declaring Bash Variables
To declare a Bash variable, you need to use the following syntax:
variable_name=value
Here, variable_name is the name of the variable, and value is the data that you want to store in the variable.
For example, to declare a local variable called myvar with the value “Hello, World!”, you would use the following command:
myvar=”Hello, World!”
To declare an environment variable called PATH with the value “/usr/local/bin:/usr/bin:/bin”, you would use the following command:
export PATH=”/usr/local/bin:/usr/bin:/bin”
Note that the export command is used to make the variable an environment variable.
Using Bash Variables
To use a Bash variable, you simply need to reference its name by prefixing it with a dollar sign ($). For example, to print the value of the myvar variable that we declared earlier, you would use the following command:
echo $myvar
This would output “Hello, World!” to the console.
You can also use variables to construct commands or parameters. For example, the following command would list all files in the /home directory:
ls /home
But if you want to list files in a specific user’s home directory, you can use the HOME environment variable like this:
ls $HOME
This would list files in the current user’s home directory.
Examples
Here are some examples of how Bash variables can be used in scripts:
1. Simple echoing of variables:
myvar=”Hello, World!”
echo $myvar
2. Concatenation of variables:
firstname=”John”
lastname=”Doe”
fullname=”$firstname $lastname”
echo $fullname
3. Use of environment variables:
echo $HOME
echo $PATH
4. Use of command-line arguments:
#!/bin/bash
echo “The first argument is $1”
echo “The second argument is $2”
In this script, $1 and $2 are special variables that hold the values of the first and second arguments passed to the script, respectively.
Conclusion
Bash variables are an essential part of Bash scripting and automation. By understanding and using them effectively, you can write powerful and flexible scripts that can save you time and effort. Whether you’re a beginner or an experienced user, we hope that this guide has been helpful in understanding Bash variables and how to use them.